1. Required tools: az and kubectl.
My host is Debian 11. First thing I need to do is to install az tool to it. According to: https://docs.microsoft.com/en-us/cli/azure/install-azure-cli-linux?pivots=apt , I chose my installation method to be the bash command below:
curl -sL https://aka.ms/InstallAzureCLIDeb | sudo bash
After the installation is complete, I have the az command available to use. I also need kubectl to access my deployed cluster from my local workstation. This is again just few commands. Some good instructions can be found from: https://kubernetes.io/docs/tasks/tools/install-kubectl-linux/ As I love one-liners, here is the command I used to get kubectl installed and ready to go on my Debian 11:
curl -LO "https://dl.k8s.io/release/$(curl -L -s https://dl.k8s.io/release/stable.txt)/bin/linux/amd64/kubectl" && chmod +x ./kubectl && sudo mv ./kubectl /usr/local/bin/kubectl
2.Beginning to make AKS cluster with the az tool.
Azure docs are great reference here: https://docs.microsoft.com/en-us/azure/aks/learn/quick-kubernetes-deploy-cli Before going any further, the login to the Azure using az tool needs to happen.
az login
“A web browser has been opened at https://login.microsoftonline.com/organizations/oauth2/v2.0/authorize. Please continue the login in the web browser. If no web browser is available or if the web browser fails to open, use device code flow with az login --use-device-code
.”
…
If I would not want to open a browser to login, I could use the -u flag
az login -u your_user@outlook.com
When navigating back to terminal, I can now see my subscription details. If I would like to change the default subscription(to another one that I might have) the commands would be:
- For listing the accounts & subscriptions:
az account list
- Setting the subscription of choice to be the default:
az account set --subscription "subscription_id_here"
When the new default subscription is selected az account list shows isDefault as true on the related details.
2.1 AKS provider dependencies
az provider show -n Microsoft.OperationsManagement -o table
az provider show -n Microsoft.OperationalInsights -o table
If the two commands, as listed above, show NotRegistered then you need to register the dependencies with:
az provider register --namespace Microsoft.OperationsManagement
az provider register --namespace Microsoft.OperationalInsights
2.2 Creating the resource group and choosing its location
For locations see this reference: https://azuretracks.com/2021/04/current-azure-region-names-reference/
az group create --name Aks --location northeurope
2.3 Creating the actual cluster with two nodes, the initial command to customize was:
az aks create -g Aks -n testAKSCluster --enable-managed-identity --node-count 2 --enable-addons monitoring --enable-msi-auth-for-monitoring --generate-ssh-keys
Managed-identities are identities managed by Azure AD.
Addons are AKS additional features that can be enabled.
Az help command gives more info on those.
az aks enable-addons -h
For example, the addons listed on the create command above have these explanations:
- monitoring: turn on Log Analytics monitoring. Requires “–workspace-resource-id”.
- –enable-msi-auth-for-monitoring : Enable Managed Identity Auth for Monitoring addon.
Since I have no need for collecting or analyzing logs on my test cluster, I will turn both of these features off.(More about Log Analytics: https://docs.microsoft.com/en-us/azure/azure-monitor/logs/log-analytics-tutorial).
Now, my command becomes: az aks create -g Aks -n testAKSCluster --enable-managed-identity --node-count 2 --generate-ssh-keys
The –generate-ssh-keys is just like it sounds: Generating keys that grant ssh access to AKS agent VMs. Notice that if the ssh keys do not already exist on your machine then they will be generated for you by the az tool.
After the az tool has done its work, I can see on the Azure UI that my AKS cluster is indeed created and running.
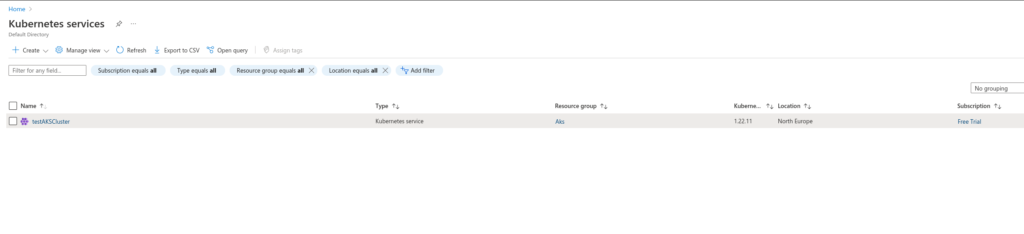
One last thing to do is to feed the AKS cluster credentials to kubectl on my workstation.
This can be achieved with: az aks get-credentials --resource-group --name
My actual command became: az aks get-credentials --resource-group Aks --name testAKSCluster
After this the az notified me that my config is at .kube/config on my home folder. Since this is the default kubeconfig location, it is possible to start building a Kubernetes environment on AKS.
Appendix:
Notice. Nodes are running with agent roles. You do not see master nodes on AKS and you shall not have access to them. AKS is a managed cluster and it gives you some added simplicity. One of these added simplicities is the case of not needing to worry about master nodes.
$ kubectl get nodes
NAME STATUS ROLES AGE VERSION
aks-nodepool1-99255722-vmss000000 Ready agent 23m v1.22.11
aks-nodepool1-99255722-vmss000001 Ready agent 23m v1.22.11
AKS pods running on my example cluster:
$ kubectl get pods --all-namespaces
NAMESPACE NAME READY STATUS RESTARTS AGE
kube-system azure-ip-masq-agent-74nz4 1/1 Running 0 26m
kube-system azure-ip-masq-agent-jcfsf 1/1 Running 0 26m
kube-system cloud-node-manager-lkv5l 1/1 Running 0 26m
kube-system cloud-node-manager-vjwww 1/1 Running 0 26m
kube-system coredns-autoscaler-7d56cd888-n5fsx 1/1 Running 0 27m
kube-system coredns-dc97c5f55-p4ncg 1/1 Running 0 27m
kube-system coredns-dc97c5f55-vflz8 1/1 Running 0 25m
kube-system csi-azuredisk-node-562hl 3/3 Running 0 26m
kube-system csi-azuredisk-node-j7dvf 3/3 Running 0 26m
kube-system csi-azurefile-node-2tjv7 3/3 Running 0 26m
kube-system csi-azurefile-node-lmn4w 3/3 Running 0 26m
kube-system konnectivity-agent-666f597486-hk2cm 1/1 Running 0 25m
kube-system konnectivity-agent-666f597486-wdppd 1/1 Running 0 27m
kube-system kube-proxy-m9xll 1/1 Running 0 26m
kube-system kube-proxy-xzldw 1/1 Running 0 26m
kube-system metrics-server-64b66fbbc8-kmcjd 1/1 Running 0 27m
Next, I am running an example deployment on AKS (please notice that this example deployment goes into default namespace, which is usually not recommended outside of a demo environment):
kubectl apply -f https://k8s.io/examples/application/deployment.yaml
After the command has applied, pods are running:
$ kubectl get pods -n default
NAME READY STATUS RESTARTS AGE
nginx-deployment-66b6c48dd5-c2zx7 1/1 Running 0 10s
nginx-deployment-66b6c48dd5-hsg5c 1/1 Running 0 10s
At this point the deployment cannot be accessed since there is no ingress-nginx controller or LoadBalancer type service configured for it.
Let’s do a quick Load Balancer service and attach it to port 80.

And then let’s create it: $ kubectl create -f mylb.yaml
service/nginx-lb created
Now we can get a new service with a Load Balancer IP (EXTERNAL-IP):
$ kubectl get svc -n default
...
nginx-lb LoadBalancer ... 20.123.106.247 80:31672/TCP 38s
When we visit the Load Balancer IP we should see the application.
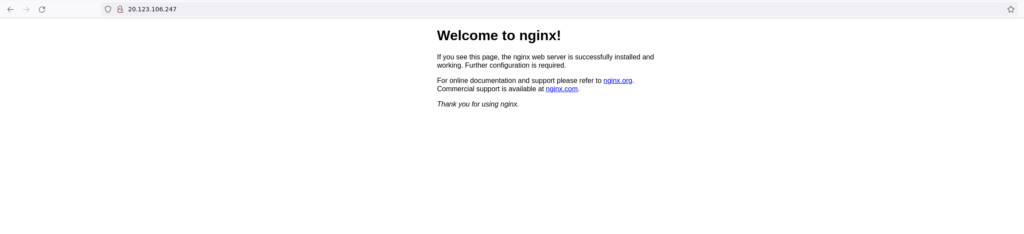
Application running on AKS.
Hint. After you are done with your daily Kubernetes fun, remember to either scale down or stop the cluster. Kubernetes is fun but it can be costly as well.